1- Butona Tıklayınca Mesaj Gösterme
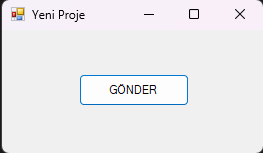
- Adım: Form üzerine görselde görüldüğü gibi bir tane Button nesnesi ekleyiniz.
- Adım: Özellikler penceresinden formun Text özelliğini “Yeni Proje” yapınız.
- Adım: Özellikler listesinden button1’in Text özelliğini “GÖNDER” yapınız.
- Adım: Olaylar listesinden button1 için Click olayı metodunu button1’in üzerine çift tıklayarak oluşturunuz.
- Adım: Oluşturduğunuz metodun içine MessageBox.Show (“Mesaj Gönderildi”); kodunu yazınız.
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show("Mesaj Gönderildi");
}
2- Butona Tıklayınca textbox nesnesine yazı yazma
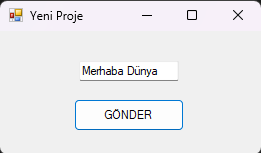
private void button1_Click(object sender, EventArgs e)
{
textBox1.Text = "Merhaba Dünya";
}
3- Tuş Takımı
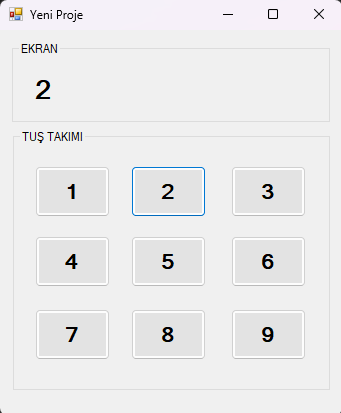
- Adım: Form üzerine Görsel 1.22’de görüldüğü gibi iki tane GroupBox nesnesi ekleyiniz.
- Adım: Üst tarafta bulunan GroupBox nesnesinin içine bir tane Label nesnesi ekleyiniz.
- Adım: Alt tarafta bulunan GroupBox nesnesinin içine on tane Button nesnesi ekleyiniz ve bunları numaralandırınız.
- Adım: Numaraların yazılı olduğu Button nesnelerinin arka plan rengini değiştirin.
- Adım: Button nesnelerinin ve Label nesnesinin yazı tipi stilini “kalın”, yazı boyutunu “20” yapınız.
- Adım: Form nesnesinin başlığını “0-9” ve Form nesnesinin arka plan rengini değiştirin.
- Adım: Tıklanan Button nesnesine ait sayıyı Label nesnesinin Text özelliğine aktaran programı yazınız.
private void button1_Click(object sender, EventArgs e)
{
label1.Text = "1";
}
private void button2_Click(object sender, EventArgs e)
{
label1.Text = "2";
}
private void button3_Click(object sender, EventArgs e)
{
label1.Text = "3";
}
private void button4_Click(object sender, EventArgs e)
{
label1.Text = "4";
}
private void button5_Click(object sender, EventArgs e)
{
label1.Text = "5";
}
private void button6_Click(object sender, EventArgs e)
{
label1.Text = "6";
}
private void button7_Click(object sender, EventArgs e)
{
label1.Text = "7";
}
private void button8_Click(object sender, EventArgs e)
{
label1.Text = "8";
}
private void button9_Click(object sender, EventArgs e)
{
label1.Text = "9";
}
4- Textbox’a Girilen Metni Label’a Yazdırma
Amaç:
Kullanıcı, bir Textbox içine yazdığı metni Label üzerinde görüntüleyebilecek.
Form Tasarımı:
- 1 adet TextBox (Kullanıcının metin girmesi için)
- 1 adet Label (Girilen metni gösterecek)
- 1 adet Button (Metni güncellemek için)
- Form Başlığı:
"Metin Güncelleme Uygulaması"
- Button’un Text özelliği:
"Güncelle"
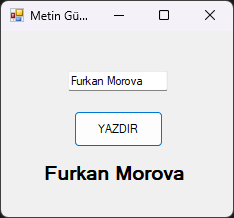
private void button1_Click(object sender, EventArgs e)
{
label1.Text = textBox1.Text; // TextBox'taki metni Label'a aktarıyor
}
5- Textbox’a girilen değeri ListBox’a ekleme
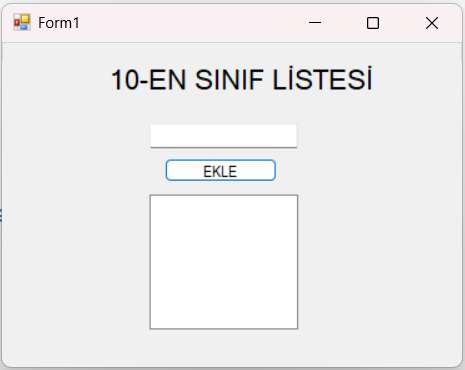
Form Tasarımı:
- 1 adet TextBox (Kullanıcının metin girmesi için)
- 1 adet Label (Başlık için)
- 1 adet Button (bastığında listeye aktarması için)
- 1 adet Listbox (eklenen verileri listelemek için)
- Button’un Text özelliği:
"Ekle"
private void button1_Click(object sender, EventArgs e)
{
string veri=textBox1.Text;
listBox1.Items.Add(veri);
}
6- C# Windows Form ile Öğrenci Kayıt Sistemi
Amaç: Birden fazla textbox’tan gelen verileri listbox’a ekleme
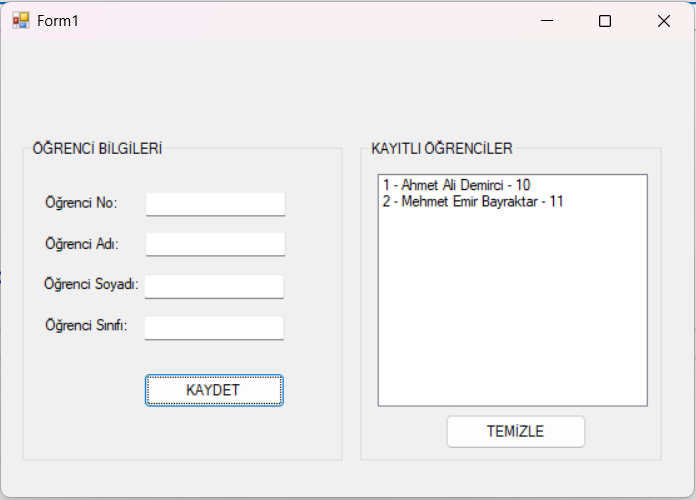
Form Tasarımı:
- 4 adet TextBox (Kullanıcının bilgileri girmesi için)
- 2 adet GroupBox
- 2 adet Button (bastığında listeye aktarması ve listeyi temizlemesi için)
- 1 adet Listbox (eklenen verileri listelemek için)
private void btnkaydet_Click(object sender, EventArgs e)
{
string ogr_no=textBox1.Text;
string ogr_ad=textBox2.Text;
string ogr_soyad=textBox3.Text;
string ogr_sinif=textBox4.Text;
listBox1.Items.Add(ogr_no+" - "+ogr_ad+" "+ogr_soyad+" - "+ogr_sinif);
textBox1.Clear();
textBox2.Clear();
textBox3.Clear();
textBox4.Clear();
}
private void btntemizle_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
}
7- C# Windows Form ile Net Hesaplama
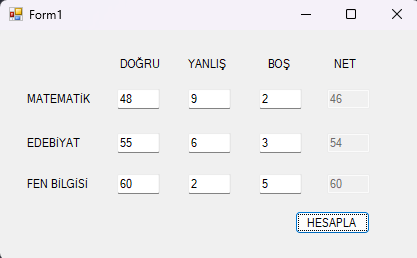
NOT: Netlerin bulunduğu textBoxların Enable özelliğini False olarak ayarlandı.
private void bhesapla_Click(object sender, EventArgs e)
{
int matD=Convert.ToInt32(textBox1.Text);
int edeD=Convert.ToInt32(textBox8.Text);
int fenD=Convert.ToInt32(textBox12.Text);
int matY=Convert.ToInt16(textBox2.Text);
int edeY=Convert.ToInt16(textBox7.Text);
int fenY=Convert.ToInt16(textBox11.Text);
float matNet = matD - (matY / 4);
float edeNet=edeD - (edeY/4);
float fenNet=fenD - (fenY/4);
textBox4.Text = matNet.ToString();
textBox5.Text = edeNet.ToString();
textBox9.Text = fenNet.ToString();
}
8- PictureBox ve RadioButton kullanarak fotoğraf geçişleri
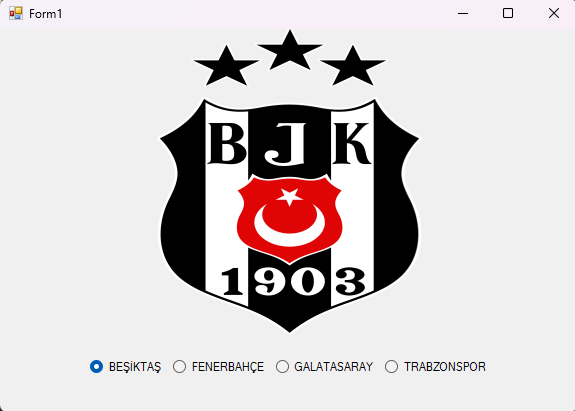
NOT: radioButtonların CheckedChanged özelliğini açmak için çift tıklamak yeterlidir.
private void radioButton1_CheckedChanged(object sender, EventArgs e)
{
pictureBox1.Visible = radioButton1.Checked;
}
private void radioButton2_CheckedChanged(object sender, EventArgs e)
{
pictureBox2.Visible = radioButton2.Checked;
}
private void radioButton3_CheckedChanged(object sender, EventArgs e)
{
pictureBox3.Visible = radioButton3.Checked;
}
private void radioButton4_CheckedChanged(object sender, EventArgs e)
{
pictureBox4.Visible = radioButton4.Checked;
}
9- Etiket Fiyatı Girilen ürünün istenilen indirim oranında indirimli fiyatını hesaplama
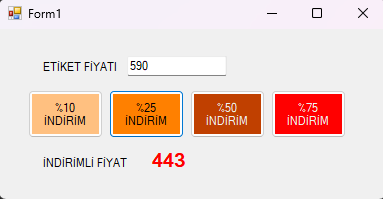
public Form1()
{
InitializeComponent();
}
int veri;
float sonuc;
private void button1_Click(object sender, EventArgs e)
{
veri=Convert.ToInt32(textBox1.Text);
sonuc=veri-(veri*10)/100;
label3.Text = sonuc.ToString();
}
private void button2_Click(object sender, EventArgs e)
{
veri = Convert.ToInt32(textBox1.Text);
sonuc = veri - (veri * 25) / 100;
label3.Text= sonuc.ToString();
}
private void button3_Click(object sender, EventArgs e)
{
veri = Convert.ToInt32(textBox1.Text);
sonuc = veri - (veri * 50) / 100;
label3.Text = sonuc.ToString();
}
private void button4_Click(object sender, EventArgs e)
{
veri = Convert.ToInt32(textBox1.Text);
sonuc = veri - (veri * 75) / 100;
label3.Text = sonuc.ToString();
}
10- Belirli Koşullara Göre Öğrenci Kayıt Sistemi
Eğer aşağıdaki koşulları karşılıyorsa listeye eklenecek. Eğer karşılamazsa MessageBox ile KAYIT BAŞARISIZ uyarısı verecek.
- Cinsiyeti Erkek olacak
- Yaşı 12’den Büyük olacak
- Temel PC Bilgisi Var olacak
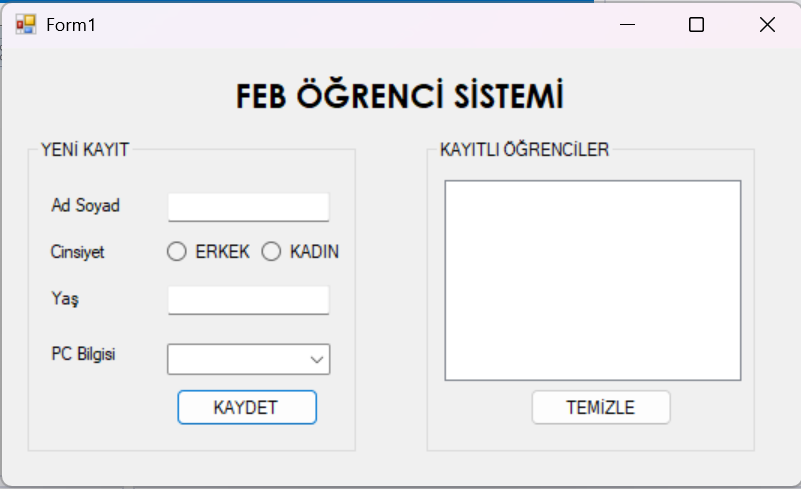
private void button1_Click(object sender, EventArgs e)
{
string ad = textBox1.Text;
int yas=Convert.ToInt32(textBox2.Text);
string pc = comboBox1.Text;
if (radioButton1.Checked == true && yas >= 12 && pc == "VAR")
{
MessageBox.Show("KAYIT BAŞARILI");
listBox1.Items.Add(ad);
}
else
{
MessageBox.Show("KAYIT BAŞARISIZ");
}
}
private void button2_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
}
11- Girilen 3 Sayıyı Büyükten Küçüğe Sıralayan Windows Form Uygulaması
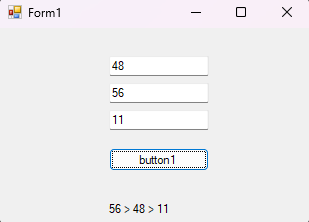
int buyuk, orta, kucuk;
private void button1_Click(object sender, EventArgs e)
{
int sayi1=Convert.ToInt32(textBox1.Text);
int sayi2=Convert.ToInt32(textBox2.Text);
int sayi3=Convert.ToInt32(textBox3.Text);
if (sayi1 > sayi2 && sayi1 > sayi3)
{
buyuk = sayi1;
if (sayi2 > sayi3)
{
orta = sayi2;
kucuk = sayi3;
}
else
{
orta = sayi3;
kucuk = sayi2;
}
}
else if (sayi2 > sayi1 && sayi2 > sayi3)
{
buyuk = sayi2;
if (sayi1 > sayi3)
{
orta=sayi1;
kucuk = sayi3;
}
else
{
orta = sayi3;
kucuk = sayi1;
}
}
else if(sayi3>sayi1 && sayi3 > sayi2)
{
buyuk=sayi3;
if (sayi1 > sayi2)
{
orta = sayi1;
kucuk = sayi2;
}
else
{
orta = sayi2;
kucuk = sayi1;
}
}
label1.Text = buyuk.ToString() + " > " + orta.ToString() + " > " + kucuk.ToString(); ;
}
12- Otel Rezervasyon Sistemi
Bu C# Windows Forms uygulaması, otel rezervasyon sistemi örneğidir. Kullanıcılar, ad-soyad, rezervasyon tarihi, oda tipi, oda numarası, konaklama süresi ve ekstra hizmetleri (örneğin yemek) seçerek bir rezervasyon oluşturabilirler.
Kullanılan Araçlar (Components)
- TextBox: Ad-soyad girişi için
- DateTimePicker: Rezervasyon tarihini seçmek için
- ComboBox: Oda tipi ve oda numarası seçimi için
- NumericUpDown: Konaklama gün sayısını belirlemek için
- CheckBox: Ekstra yemek seçeneği için
- Label: Toplam ücreti göstermek için
- Button: Rezervasyonu tamamlamak için
- ListBox veya RichTextBox: Yapılan rezervasyonları listelemek için
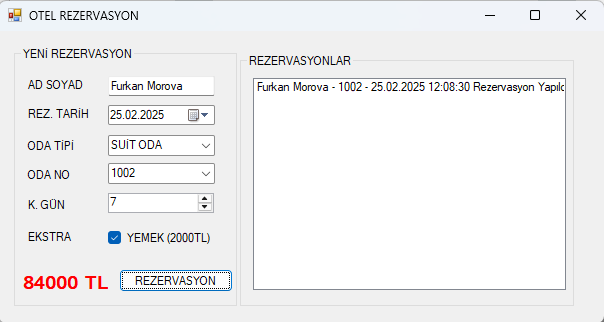
int ucret = 0;
private void button1_Click(object sender, EventArgs e)
{
//Verileri kullanıcıdan çektim
string adsoyad=textBox1.Text;
DateTime tarih=dateTimePicker1.Value;
string odatip=comboBox1.Text;
string odano=comboBox2.Text;
int gun=(int)numericUpDown1.Value;
if (odatip == "TEK KİŞİLİK")
{
ucret += 3000;
}
else if (odatip == "ÇİFT KİŞİLİK")
{
ucret += 5000;
}
else
{
ucret += 10000;
}
if (checkBox1.Checked == true)
{
ucret = (gun * 2000) + (gun * ucret);
label7.Text= ucret.ToString()+ " TL";
}
else {
ucret = (gun * ucret);
label7.Text = ucret.ToString() + " TL";
}
MessageBox.Show("Rezervasyon Başarılı");
listBox1.Items.Add(adsoyad+" - "+odano+" - "+tarih+" Rezervasyon Yapıldı.");
}
13- İki Tarih Arası Gün Farkı Hesaplama
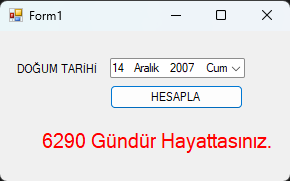
private void button1_Click(object sender, EventArgs e)
{
DateTime dogum=dateTimePicker1.Value;
//DateTime.Now Bugünün Tarihini verir.
DateTime bugun=DateTime.Now;
// TimeSpan İki tarih arasındaki farkı hesaplar
TimeSpan fark = bugun - dogum;
// fark.days: Farkı güne çevirir.
int gun=fark.Days;
label2.Text= gun.ToString()+" Gündür Hayattasınız.";
}
14- C# Windows Form Button ile Sayaç Kullanımı
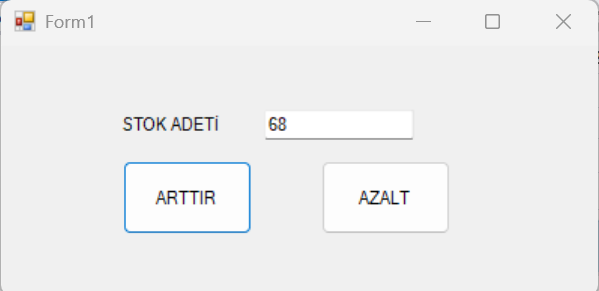
int sayi;
private void button1_Click(object sender, EventArgs e)
{
sayi = Convert.ToInt32(textBox1.Text);
sayi += 1;
textBox1.Text = sayi.ToString();
}
private void button2_Click(object sender, EventArgs e)
{
sayi = Convert.ToInt32(textBox1.Text);
sayi -= 1;
textBox1.Text = sayi.ToString();
}
15- PictureBox’ı Button ile İleri Geri Hareket Ettirme
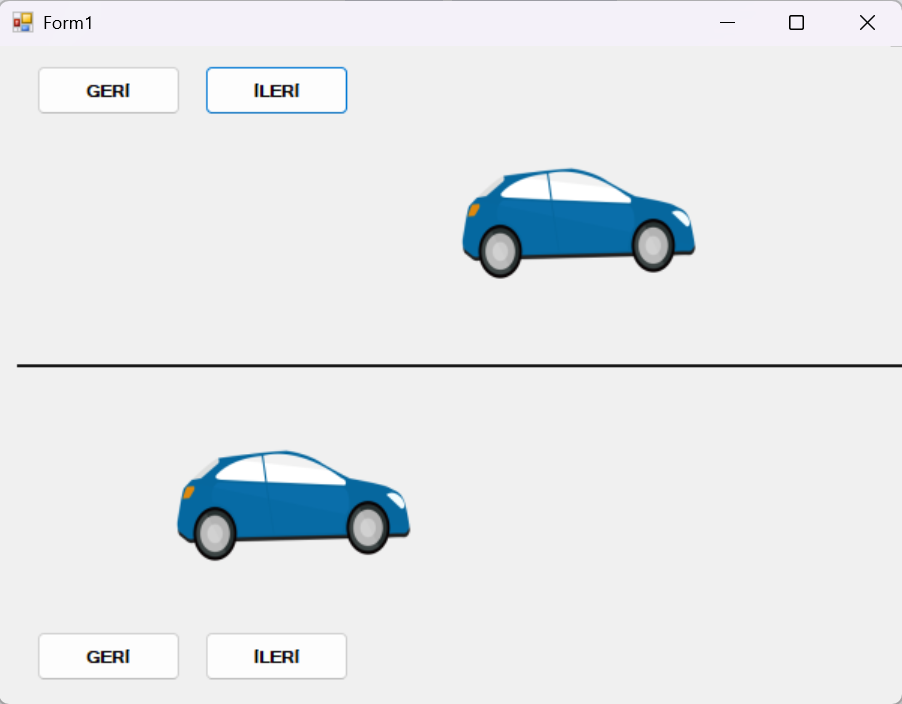
private void araba1ileri_Click(object sender, EventArgs e)
{
pictureBox1.Left = pictureBox1.Left + 10;
}
private void araba1geri_Click(object sender, EventArgs e)
{
pictureBox1.Left = pictureBox1.Left - 10;
}
private void araba2geri_Click(object sender, EventArgs e)
{
pictureBox2.Left = pictureBox2.Left - 10;
}
private void araba2ileri_Click(object sender, EventArgs e)
{
pictureBox2.Left = pictureBox2.Left + 10;
}
16- C# Windows Form Dizi Örneği
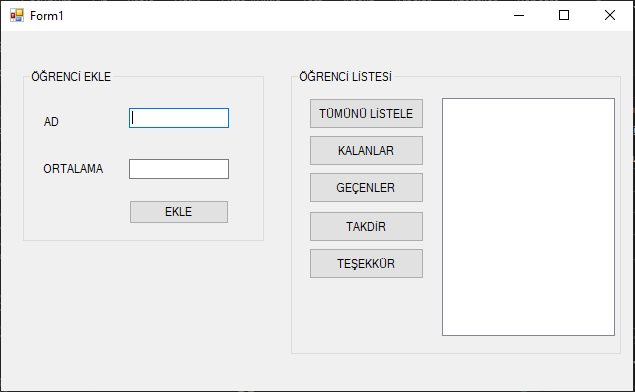
string[] ogr_isim = new string[10];
int[] ogr_ort = new int[10];
int indeks = 0;
private void ekle_Click(object sender, EventArgs e)
{
ogr_isim[indeks] = textBox1.Text;
ogr_ort[indeks] = Convert.ToInt32(textBox2.Text);
indeks++;
textBox1.Clear();
textBox2.Clear();
if (indeks == 10)
{
button6.Enabled = false;
}
}
private void tumunulistele_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
for(int i = 0; i < ogr_isim.Length; i++)
{
listBox1.Items.Add(ogr_isim[i] + " " + ogr_ort[i]);
}
}
private void kalanlar_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
for(int i = 0; i < ogr_ort.Length; i++)
{
if (ogr_ort[i] < 50)
{
listBox1.Items.Add(ogr_isim[i] + " " + ogr_ort[i]);
}
}
}
private void gecenler_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
for(int i = 0; i < ogr_ort.Length; i++)
{
if (ogr_ort[i] >= 50)
{
listBox1.Items.Add(ogr_isim[i] + " " + ogr_ort[i]);
}
}
}
private void takdir_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
for (int i = 0; i < ogr_ort.Length; i++)
{
if (ogr_ort[i] >= 85)
{
listBox1.Items.Add(ogr_isim[i] + " " + ogr_ort[i]);
}
}
}
private void tesekkur_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
for (int i = 0; i < ogr_ort.Length; i++)
{
if (ogr_ort[i] >= 70 && ogr_ort[i]<85)
{
listBox1.Items.Add(ogr_isim[i] + " " + ogr_ort[i]);
}
}
}
17- C# Windows Form CheckBox kullanarak hesap makinesi
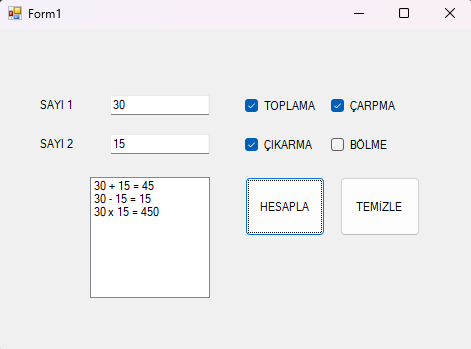
private void hesapla_Click(object sender, EventArgs e)
{
int sayi1 = Convert.ToInt16(textBox1.Text);
int sayi2 = Convert.ToInt16(textBox2.Text);
int islem;
if (checkBox1.Checked == true)
{
islem = sayi1 + sayi2;
listBox1.Items.Add($"{sayi1} + {sayi2} = {islem}");
}
if (checkBox2.Checked == true)
{
islem = sayi1 - sayi2;
listBox1.Items.Add($"{sayi1} - {sayi2} = {islem}");
}
if (checkBox3.Checked == true)
{
islem = sayi1 / sayi2;
listBox1.Items.Add($"{sayi1} / {sayi2} = {islem}");
}
if (checkBox4.Checked == true)
{
islem = sayi1 * sayi2;
listBox1.Items.Add($"{sayi1} x {sayi2} = {islem}");
}
}
private void temizle_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
}
18- C# Windows Form ile Vize ve Final Notu girilen öğrencinin ortalamasını ve harf notunu hesaplama
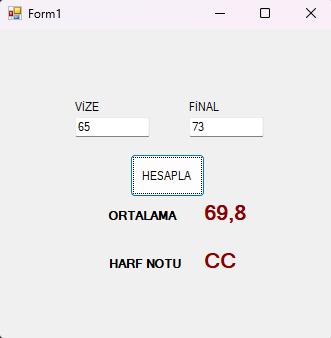
private void button1_Click(object sender, EventArgs e)
{
int vize = Convert.ToInt16(textBox1.Text);
int final = Convert.ToInt16(textBox2.Text);
double ortalama = (vize * 0.40) + (final * 0.60);
label5.Text = ortalama.ToString();
if (ortalama <= 100)
{
if (ortalama >= 90)
{
label6.Text = "AA";
}
else if (ortalama >= 85)
{
label6.Text = "BA";
}
else if (ortalama >= 80)
{
label6.Text = "BB";
}
else if (ortalama >= 70)
{
label6.Text = "CB";
}
else if (ortalama >= 60)
{
label6.Text = "CC";
}
else if (ortalama >= 55)
{
label6.Text = "DC";
}
else if (ortalama >= 50)
{
label6.Text = "DD";
}
else if (ortalama >= 40)
{
label6.Text = "FD";
}
else if (ortalama >= 0)
{
label6.Text = "FF";
}
}
else
{
MessageBox.Show("HATA");
}
}
19- C# Windows Form ile Döngü Kullanımı
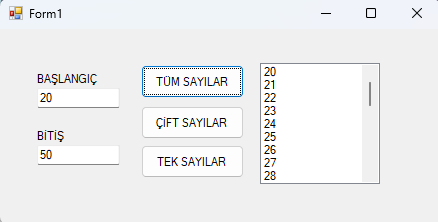
private void tumubtn_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
int sayi1 = Convert.ToInt32(textBox1.Text);
int sayi2 = Convert.ToInt32(textBox2.Text);
for(int i = sayi1; i <= sayi2; i++)
{
listBox1.Items.Add(i);
}
}
private void ciftbtn_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
int sayi1 = Convert.ToInt32(textBox1.Text);
int sayi2 = Convert.ToInt32(textBox2.Text);
for (int i = sayi1; i <= sayi2; i++)
{
if (i % 2 == 0)
{
listBox1.Items.Add(i);
}
}
}
private void tekbtn_Click(object sender, EventArgs e)
{
listBox1.Items.Clear();
int sayi1 = Convert.ToInt32(textBox1.Text);
int sayi2 = Convert.ToInt32(textBox2.Text);
for (int i = sayi1; i <= sayi2; i++)
{
if (i % 2 == 1)
{
listBox1.Items.Add(i);
}
}
}